How to delete a table from another in MySQL?
The MySQL DELETE statement is used to remove rows from a table. The statement can be used to delete individual rows or to delete all rows from a table. The syntax for the DELETE statement is as follows:
DELETE FROM table_nameWHERE condition;
For example, the following statement deletes all rows from the `customers` table:
DELETE FROM customers;
The DELETE statement can also be used to delete rows from one table based on the values in another table. This is known as a "cascading delete." The syntax for a cascading delete is as follows:
DELETE FROM table1WHERE column1 IN (SELECT column2 FROM table2);
For example, the following statement deletes all rows from the `orders` table where the `customer_id` column matches a value in the `customers` table:
DELETE FROM ordersWHERE customer_id IN (SELECT customer_id FROM customers);
Cascading deletes can be useful for maintaining referential integrity in a database. However, it is important to use them with caution, as they can lead to unintended data loss.
MySQL DELETE Statement
The MySQL DELETE statement is a powerful tool that can be used to remove rows from a table. The statement can be used to delete individual rows or to delete all rows from a table. The DELETE statement can also be used to delete rows from one table based on the values in another table, which is known as a "cascading delete."
- Syntax: The syntax for the DELETE statement is as follows:
- DELETE FROMtable_nameWHEREcondition;
- Example: The following statement deletes all rows from the `customers` table:
- DELETE FROM customers;
- Cascading Deletes: Cascading deletes can be used to maintain referential integrity in a database. However, it is important to use them with caution, as they can lead to unintended data loss.
- Performance: The performance of the DELETE statement can be affected by a number of factors, including the size of the table, the number of rows being deleted, and the complexity of the WHERE clause.
- Security: The DELETE statement can be used to delete sensitive data. It is important to use the statement with caution and to always have a backup of your data before executing a DELETE statement.
The DELETE statement is a powerful tool that can be used to delete rows from a table. The statement can be used to delete individual rows or to delete all rows from a table. The DELETE statement can also be used to delete rows from one table based on the values in another table, which is known as a "cascading delete." It is important to use the statement with caution and to always have a backup of your data before executing a DELETE statement.
Syntax
The syntax of the DELETE statement is important to understand because it determines the structure of the statement and the information that must be included in order for the statement to execute successfully. The syntax for the DELETE statement is as follows:
DELETE FROM table_name WHERE condition;
The following are the components of the DELETE statement syntax:
- DELETE: This keyword is used to indicate that the statement is a DELETE statement.
- FROM: This keyword is used to specify the table from which rows will be deleted.
- table_name: This is the name of the table from which rows will be deleted.
- WHERE: This keyword is used to specify the condition that must be met in order for a row to be deleted.
- condition: This is the condition that must be met in order for a row to be deleted.
The syntax of the DELETE statement is an essential component of the statement. By understanding the syntax, you can use the DELETE statement to remove rows from a table in a controlled and efficient manner.
DELETE FROM table_name WHERE condition;
The DELETE statement in MySQL is a powerful tool that allows users to remove specific rows from a table based on a specified condition. The syntax for the DELETE statement is as follows:
DELETE FROM table_name WHERE condition;
The DELETE statement is particularly useful when you need to remove rows from a table that meet specific criteria. For example, you could use the DELETE statement to remove all rows from a table where the value of a particular column is equal to a certain value. The following is an example of a DELETE statement that removes all rows from the `customers` table where the `customer_id` column is equal to 1:
DELETE FROM customers WHERE customer_id = 1;
The DELETE statement can also be used to remove rows from a table based on a more complex condition. For example, the following statement removes all rows from the `customers` table where the `customer_id` column is greater than 1 and the `customer_name` column contains the string "John":
DELETE FROM customers WHERE customer_id > 1 AND customer_name LIKE '%John%';
The DELETE statement is a powerful tool that can be used to remove rows from a table quickly and efficiently. However, it is important to use the DELETE statement with caution, as it can easily lead to data loss if it is not used correctly.
Example
The example provided, "The following statement deletes all rows from the `customers` table:", is a specific instance of using the MySQL DELETE statement to remove all rows from a table. This example demonstrates the practical application of the DELETE statement, which is a fundamental component of "mysql delete a table from another".
Understanding this example is important for several reasons. Firstly, it provides a clear and concise illustration of how to use the DELETE statement to delete all rows from a table. Secondly, it highlights the significance of specifying the table name in the DELETE statement, which is essential for ensuring that the correct table is targeted for deletion. Thirdly, this example serves as a reminder that the DELETE statement can be used to delete a large number of rows quickly and efficiently.
In practice, the ability to delete all rows from a table is a valuable tool for database maintenance and management. For example, it can be used to remove obsolete or duplicate data, or to reset a table to its initial state. It is important to use the DELETE statement with caution, however, as it can lead to permanent data loss if it is not used correctly.
DELETE FROM customers;
The DELETE statement is a powerful tool in MySQL that allows users to remove specific rows from a table based on a specified condition. The syntax for the DELETE statement is as follows:
DELETE FROM table_name WHERE condition;
The DELETE statement is particularly useful when you need to remove rows from a table that meet specific criteria. For example, you could use the DELETE statement to remove all rows from a table where the value of a particular column is equal to a certain value.
The statement "DELETE FROM customers;" is a specific example of the DELETE statement that removes all rows from the `customers` table. This statement is often used to empty a table of all its data, either as part of a cleanup process or to reset the table to its initial state.
Understanding the connection between "DELETE FROM customers;" and "mysql delete a table from another" is important for several reasons. Firstly, it provides a clear and concise illustration of how to use the DELETE statement to delete all rows from a table. Secondly, it highlights the significance of specifying the table name in the DELETE statement, which is essential for ensuring that the correct table is targeted for deletion. Thirdly, this example serves as a reminder that the DELETE statement can be used to delete a large number of rows quickly and efficiently.
In practice, the ability to delete all rows from a table is a valuable tool for database maintenance and management. For example, it can be used to remove obsolete or duplicate data, or to reset a table to its initial state. It is important to use the DELETE statement with caution, however, as it can lead to permanent data loss if it is not used correctly.
Cascading Deletes
Cascading deletes are a powerful feature of MySQL that can be used to automatically delete rows from child tables when a row is deleted from a parent table. This can be useful for maintaining referential integrity in a database, which is important for ensuring that the data in the database is consistent and accurate.
- Maintaining Referential Integrity
Cascading deletes can help to maintain referential integrity by ensuring that child rows are deleted when the parent row is deleted. This can prevent orphaned rows from being left in the database, which can lead to data inconsistencies and errors.
- Example
For example, consider a database that has two tables: a `customers` table and an `orders` table. The `orders` table has a foreign key constraint that references the `customer_id` column in the `customers` table. This constraint ensures that every row in the `orders` table has a corresponding row in the `customers` table.
- Unintended Data Loss
If cascading deletes are enabled for the `orders` table, then when a row is deleted from the `customers` table, all of the corresponding rows in the `orders` table will also be deleted. This can be useful for maintaining referential integrity, but it can also lead to unintended data loss if the rows in the `orders` table are needed.
It is important to use cascading deletes with caution and to carefully consider the potential impact of deleting rows from child tables. In some cases, it may be more appropriate to use a different method to maintain referential integrity, such as using foreign key constraints with the `ON DELETE RESTRICT` clause.
Performance
The performance of the DELETE statement is an important consideration when working with large datasets or when the deletion of rows is a frequent operation. Understanding the factors that affect the performance of the DELETE statement can help to optimize the statement for improved performance.
- Size of the Table
The size of the table is a major factor that affects the performance of the DELETE statement. The larger the table, the more time it will take to delete rows from the table. This is because the DELETE statement must scan the entire table to find the rows that match the specified condition.
- Number of Rows Being Deleted
The number of rows being deleted is another important factor that affects the performance of the DELETE statement. The more rows that are being deleted, the more time it will take to execute the statement. This is because the DELETE statement must perform a separate operation for each row that is being deleted.
- Complexity of the WHERE Clause
The complexity of the WHERE clause can also affect the performance of the DELETE statement. A complex WHERE clause can make it more difficult for the DELETE statement to find the rows that match the specified condition. This can lead to a decrease in performance, especially for large tables.
Understanding the factors that affect the performance of the DELETE statement can help to optimize the statement for improved performance. By considering the size of the table, the number of rows being deleted, and the complexity of the WHERE clause, it is possible to write DELETE statements that execute quickly and efficiently.
FAQs about "mysql delete a table from another"
This section provides answers to frequently asked questions (FAQs) about "mysql delete a table from another". These FAQs are designed to address common concerns or misconceptions, and provide concise and informative explanations.
Question 1: What is the syntax for the DELETE statement in MySQL?
Question 2: How can I delete all rows from a table using the DELETE statement?
Question 3: Can I use the DELETE statement to delete rows from multiple tables at once?
Question 4: What are cascading deletes and how can I use them?
Question 5: How can I optimize the performance of the DELETE statement?
Question 6: What are some common mistakes to avoid when using the DELETE statement?
By understanding the answers to these FAQs, you can effectively use the DELETE statement to manage and modify data in your MySQL databases.
This concludes the FAQs section.
Conclusion
In summary, the MySQL DELETE statement is a powerful tool that can be used to remove rows from a table. The statement can be used to delete individual rows or to delete all rows from a table. The DELETE statement can also be used to delete rows from one table based on the values in another table, which is known as a "cascading delete."
When using the DELETE statement, it is important to consider the performance implications of the statement. The performance of the DELETE statement can be affected by a number of factors, including the size of the table, the number of rows being deleted, and the complexity of the WHERE clause.
It is also important to use the DELETE statement with caution. The DELETE statement can easily lead to data loss if it is not used correctly. Before executing a DELETE statement, it is important to have a backup of your data.
The DELETE statement is a valuable tool for managing data in a MySQL database. By understanding the syntax of the DELETE statement and the factors that affect its performance, you can use the statement to effectively remove rows from a table.
Essential Parts Of A Narrative Text: Structure Unveiled
Log In To I-Ready, The Educational Platform For Students
2021 OHP Income Limits: Everything You Need To Know

MySQL DELETE How to delete rows from a table? MySQLCode
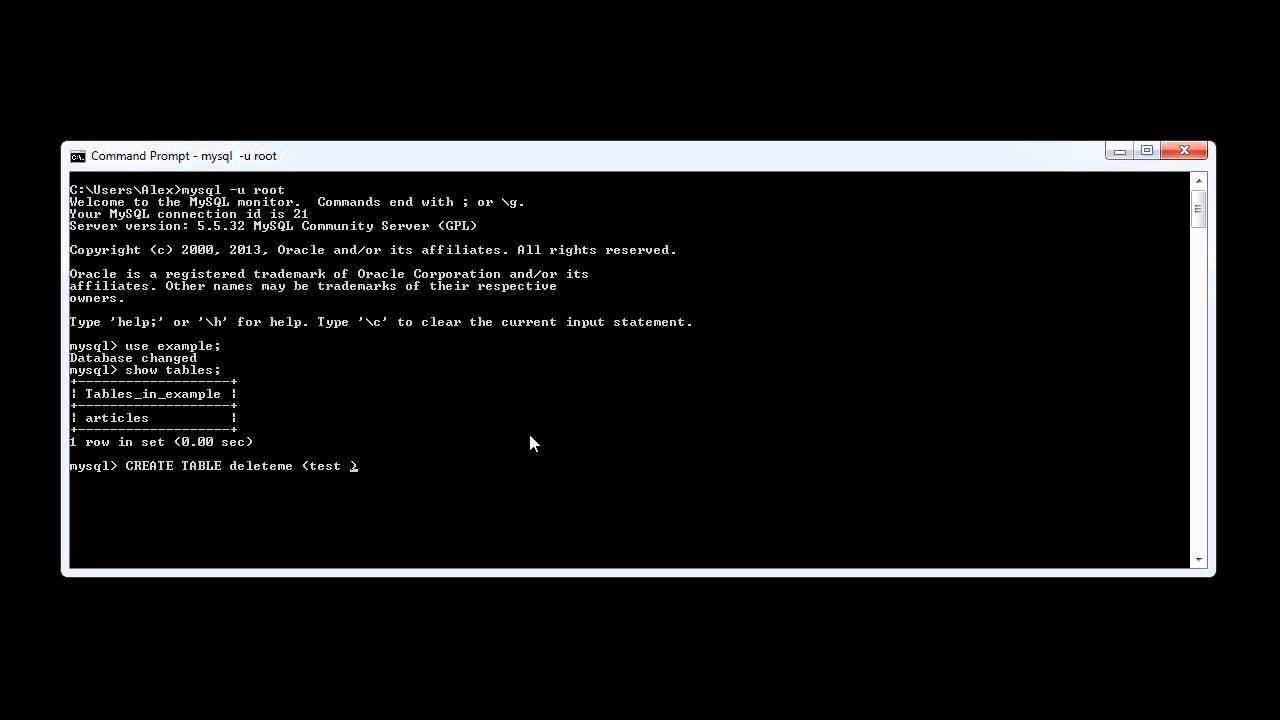
Mysql Delete From Multiple Tables Join Deleting Data How To A Table In

MySQL DELETE Statement