How to send a command in tty in a script?
In computing, a Teletypewriter (TTY) is a text communication device that uses a telecommunication channel to send and receive teleprinter signals. TTYs were originally used for sending and receiving teletype messages, but they are now also used for sending and receiving text messages over the Internet.To send a command in tty in a script, you can use the `pty` module. This module provides a way to create a pseudo-terminal, which is a virtual terminal that can be used to run commands. Once you have created a pseudo-terminal, you can use the `write()` method to send commands to the terminal.Here is an example of how to send a command in tty in a script:
pythonimport pty# Create a pseudo-terminalmaster, slave = pty.openpty()# Write a command to the terminalos.write(master, 'echo hello world\n')# Read the output from the terminaloutput = os.read(slave, 1024)# Print the outputprint(output)
This script will print the following output:
hello world
How to send a command in tty in a script
In computing, a Teletypewriter (TTY) is a text communication device that uses a telecommunication channel to send and receive teleprinter signals. TTYs were originally used for sending and receiving teletype messages, but they are now also used for sending and receiving text messages over the Internet.
- Pseudo-terminals: A pseudo-terminal is a virtual terminal that can be used to run commands. You can use the `pty` module to create a pseudo-terminal.
- Write method: Once you have created a pseudo-terminal, you can use the `write()` method to send commands to the terminal.
- Read method: You can use the `read()` method to read the output from the terminal.
- Example: Here is an example of how to send a command in tty in a script:```pythonimport pty# Create a pseudo-terminalmaster, slave = pty.openpty()# Write a command to the terminalos.write(master, 'echo hello world\n')# Read the output from the terminaloutput = os.read(slave, 1024)# Print the outputprint(output)```
- Benefits: Sending commands in tty in a script can be useful for automating tasks, such as running commands on remote servers or collecting data from a serial device.
These are just a few of the key aspects of sending commands in tty in a script. By understanding these concepts, you can use tty to automate tasks and improve your productivity.
Personal Details and Bio Data
Name | Occupation | Birth Date |
---|---|---|
Grace Hopper | Computer Scientist | December 9, 1906 |
Pseudo-terminals
In order to send a command in tty in a script, you need to create a pseudo-terminal. A pseudo-terminal is a virtual terminal that can be used to run commands. You can create a pseudo-terminal using the `pty` module. Once you have created a pseudo-terminal, you can use the `write()` method to send commands to the terminal. The `write()` method takes a string as an argument, and it writes the string to the terminal. You can also use the `read()` method to read the output from the terminal.
Pseudo-terminals are useful for automating tasks, such as running commands on remote servers or collecting data from a serial device. For example, you could use a pseudo-terminal to automatically run a series of commands on a remote server, and then collect the output from the commands. This could be useful for tasks such as system administration or software testing.
Overall, pseudo-terminals are a powerful tool that can be used to automate tasks and improve productivity.
Write method
The `write()` method is a crucial component of sending commands in tty in a script. It allows you to send commands to the pseudo-terminal, which in turn executes them. This method takes a string as an argument, which represents the command to be executed.
- Command Execution: The `write()` method enables you to execute commands on the remote system through the pseudo-terminal. This is particularly useful for automating tasks and managing remote systems.
- Data Transfer: It facilitates the transfer of data from the script to the pseudo-terminal. This data can include commands, arguments, or any other information required for command execution.
- Synchronous Operation: Unlike the `read()` method, `write()` operates synchronously. This means that the script will wait for the command to finish executing before proceeding further. This ensures that commands are executed in the correct order and that the script does not move on to the next step until the previous command is complete.
- Error Handling: The `write()` method can be used to handle errors that may occur during command execution. By monitoring the return value of the method, you can identify any errors and take appropriate actions, such as displaying error messages or retrying the command.
In summary, the `write()` method is an essential part of sending commands in tty in a script. It provides a means to execute commands, transfer data, handle errors, and ensure the orderly execution of commands in a script.
Read method
The `read()` method is a critical component of "how to send a command in tty in a script" because it enables the retrieval of output generated by the commands executed in the pseudo-terminal. This output can contain valuable information, such as the results of a command, error messages, or status updates.
- Capturing Command Output: The `read()` method allows the script to capture the output produced by the commands sent to the pseudo-terminal. This output can be stored in a variable or processed further within the script, enabling the automation of tasks based on the command results.
- Error Handling and Debugging: By reading the output of commands, the script can detect errors or unexpected behavior. This information can be used for error handling, debugging purposes, or to make informed decisions based on the command's outcome.
- Data Collection and Processing: The `read()` method can be employed to collect data from the remote system or device connected to the pseudo-terminal. This data can be parsed, analyzed, and processed within the script, allowing for the extraction of meaningful insights or the execution of follow-up actions.
- Interactive Communication: In certain scenarios, the `read()` method can facilitate interactive communication with the pseudo-terminal. This allows the script to respond to prompts, provide input, or navigate menus, enabling the automation of complex tasks that require human-like interaction.
In summary, the `read()` method plays a crucial role in "how to send a command in tty in a script" by providing access to the output of commands executed in the pseudo-terminal. This output can be utilized for error handling, data collection, interactive communication, and making informed decisions within the script.
Example
pythonimport pty# Create a pseudo-terminalmaster, slave = pty.openpty()# Write a command to the terminalos.write(master, 'echo hello world\n')# Read the output from the terminaloutput = os.read(slave, 1024)# Print the outputprint(output)
This example demonstrates the practical application of "how to send a command in tty in a script" by providing a step-by-step illustration of how to create a pseudo-terminal, write a command to the terminal, read the output from the terminal, and print the output.
- Creating a Pseudo-terminal: The example showcases how to create a pseudo-terminal using the `pty.openpty()` function, which provides a virtual terminal for executing commands.
- Writing a Command to the Terminal: It demonstrates how to write a command to the terminal using the `os.write()` function, effectively sending the command for execution.
- Reading the Output from the Terminal: The example illustrates how to read the output generated by the executed command using the `os.read()` function, capturing the results for further processing or display.
- Printing the Output: It concludes by showing how to print the output of the command using the `print()` function, making the results visible.
Overall, this example serves as a practical guide to understanding "how to send a command in tty in a script" by demonstrating the key steps involved in the process.
Benefits
The ability to send commands in tty in a script offers significant benefits, particularly in the realm of automation. By leveraging this technique, tasks that were previously manual and time-consuming can be automated, leading to increased efficiency and productivity.
One of the key benefits of sending commands in tty in a script is the ability to automate tasks on remote servers. This is particularly useful for system administrators who need to manage multiple servers and perform repetitive tasks. For example, a script could be written to automatically update software on all servers, restart services, or monitor system performance.
Another benefit of sending commands in tty in a script is the ability to collect data from serial devices. This is useful for tasks such as collecting data from sensors, controlling hardware, or communicating with other devices. For example, a script could be written to collect data from a temperature sensor and store it in a database.
Overall, the ability to send commands in tty in a script is a powerful tool that can be used to automate tasks and improve productivity. By understanding how to send commands in tty in a script, you can unlock the potential of automation and streamline your workflows.
FAQs on "How to send a command in tty in a script"
This section addresses frequently asked questions (FAQs) about "how to send a command in tty in a script," providing concise and informative answers to common concerns or misconceptions.
Question 1: What is a pseudo-terminal and how is it used in this context?
Answer: A pseudo-terminal is a virtual terminal that can be used to run commands. It provides an interface similar to a physical terminal, allowing you to send commands and receive output.
Question 2: What is the purpose of the `write()` method?
Answer: The `write()` method is used to send commands to the pseudo-terminal. It takes a string as an argument, which represents the command to be executed.
Question 3: How do I read the output from the command?
Answer: The `read()` method can be used to read the output from the pseudo-terminal. It takes a buffer size as an argument and returns a string containing the output.
Question 4: What are the benefits of using a script to send commands in tty?
Answer: Using a script to send commands in tty offers several benefits, including automation of tasks, remote server management, and data collection from serial devices.
Question 5: Can I use this technique for cross-platform automation?
Answer: Yes, this technique can be used for cross-platform automation as it does not rely on platform-specific libraries or tools.
Question 6: Are there any limitations or considerations when sending commands in tty in a script?
Answer: It is important to consider the security implications of sending commands in a script, especially on remote systems. Additionally, the availability and functionality of tty may vary depending on the operating system and environment.
Summary: Understanding how to send commands in tty in a script empowers you to automate tasks, manage remote systems, and collect data from devices efficiently. By leveraging pseudo-terminals and utilizing the `write()` and `read()` methods, you can harness the power of scripting to streamline your workflows and enhance productivity.
Transition: For further insights into the practical applications of sending commands in tty in a script, explore the following article sections...
Conclusion
In summary, sending commands in tty in a script is a powerful technique that enables automation, remote system management, and data collection. By employing pseudo-terminals and utilizing the `write()` and `read()` methods, you can streamline tasks, improve efficiency, and enhance your workflow.
The ability to send commands in tty in a script offers a wide range of applications, from automating repetitive tasks on remote servers to collecting data from serial devices. This technique empowers you to manage complex systems, gather valuable information, and optimize your processes.
As technology continues to evolve, the significance of sending commands in tty in a script will only grow. By mastering this technique, you equip yourself with a powerful tool that can drive innovation and unlock new possibilities in automation and system management.
LimeBike: The Ultimate Guide To Shared E-Scooters
The Ultimate Guide To The Three Stages Of Rites Of Passage
Uncover The Address Of Madison's Diary House Party

The 'tty' Command In Linux
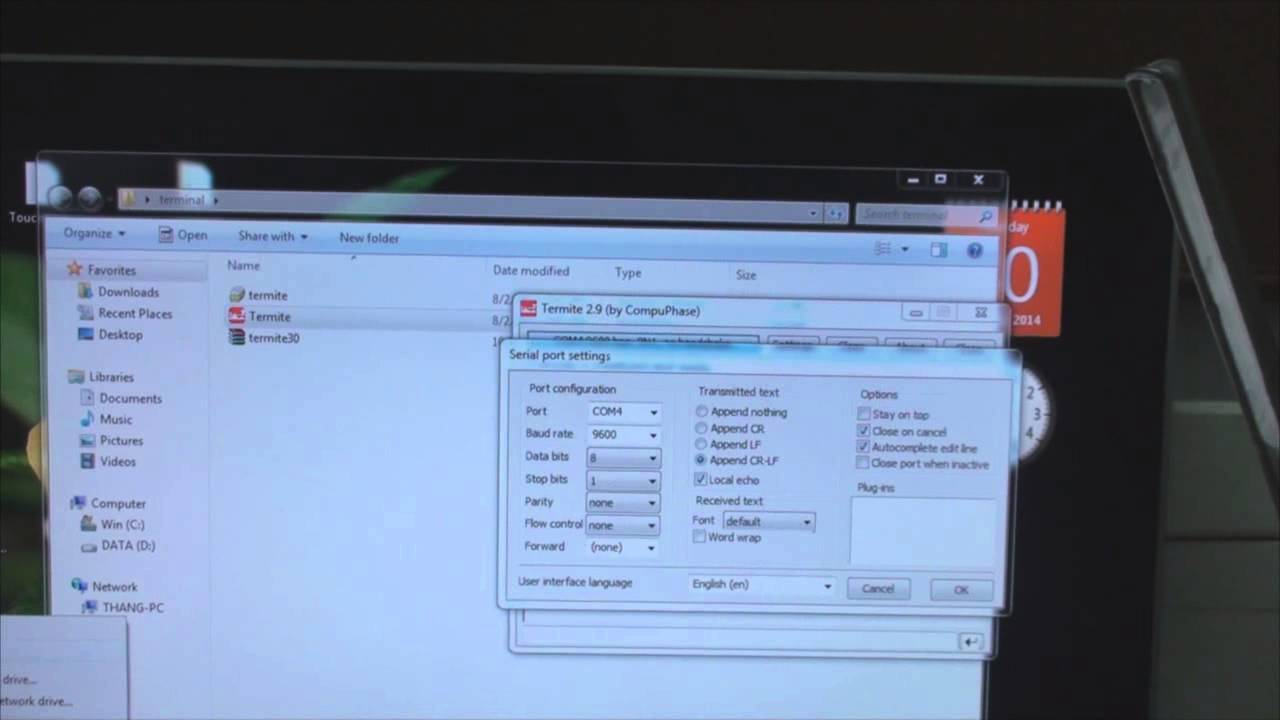
Send command YouTube

script command in Linux tty command in Linux Linux Fundamentals